Git is a powerful version control system that allows developers to manage their codebase effectively. While many developers use Git on a daily basis, there are several lesser-known but useful Git commands and features that can make your workflow more efficient. Letβs explore some of the most commonly used commands across different stages of a project.
Table of Contents
Setup and Config
git config
Configuring configuration parameters for a Git installation is possible with the git config command. This is crucial in order to customize the behavior of Git to your specific preferences. Whether you wish to review the current configurations or set your name and email address globally for all repositories, git config
has you covered.
π οΈ Handy Options:
--global
: Sets configurations globally, ensuring consistency across all repositories.--list
: Lists all configurations, providing an overview of the current settings.
π‘Example:
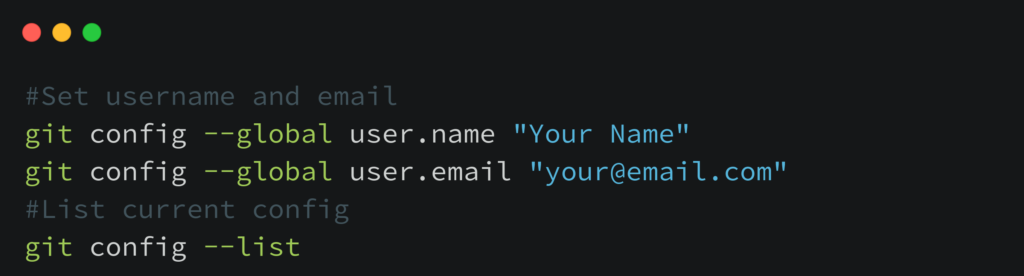
#Set alias for command
git config --global alias.<-aliasname-> <-commandname->
#Setting the default editor for Git
git config --global core.editor "vim"
Getting and Creating Project
git init
git init
initializes a new Git repository in the current directory, enabling version control for your project.
π‘Example:
git init
git clone
git clone
creates a copy of an existing Git repository, including all branches and history.
π οΈ Handy Options:
--depth <depth>
: Clones only the specified number of commits from the remote repository.--branch <branch>
: Clones only a specific branch.
π‘Example:
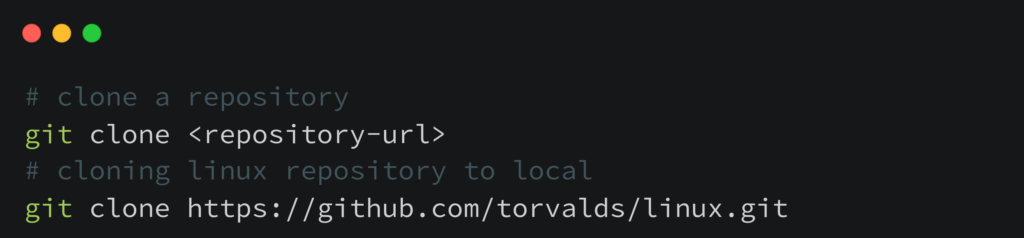
git clone --depth 1 --branch main <-repository-url->
Basic Snapshotting
git add
git add
stages changes for commit. It prepares files to be included in the next commit snapshot.
π οΈ Handy Options:
-A
or--all
: Adds all changes to the staging area.<file>
: Adds specific files to the staging area.
π‘Example:
git add .
git add <-file->
git commit
git commit
records changes to the repository, creating a new commit with a commit message.
π οΈ Handy Options:
-m "<message>"
: Adds a commit message inline.
π‘Example:
git commit -m "Initial commit"
git status
The git status
command gives a complete summary of the repository’s present condition. It provides information regarding staged and unstaged modifications, in addition to tracked and untracked file status. This command is essential for determining which changes have been made and which remain to be committed.
π‘Example:
git status
# git status on libgit2 repo
β libgit2 git:(main) git status
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
git diff
The git diff
command is used to examine the distinctions among different repository states, including modifications made between the staging area and the working directory, as well as between commits. It offers an extensive analysis of modifications, which helps with insight of the modifications applied on files.
π‘Example:
git diff
git reset
The git reset
command enables the reversal of repository modifications through the restoration of the current HEAD to a predetermined state. By doing so, modifications can be undone, the HEAD can be shifted to an alternative commit, or the working directory can be restored to correspond with a particular commit.
π οΈ Handy Options:
--soft
: Resets the HEAD to a specified commit, keeping the changes staged.--mixed
: Resets the HEAD to a specified commit and unstages changes, but preserves the changes in the working directory.--hard
: Resets the HEAD to a specified commit and discards all changes in the working directory.
π‘Example:
git reset --soft HEAD~1
git reset --mixed HEAD~1
git reset --hard HEAD~1
Branching and Merging
git branch
git branch
lists existing branches, and with additional options, it can create or delete branches.
π οΈ Handy Options:
-a
or--all
: Lists both remote-tracking and local branches.-d <branch>
: Deletes the specified branch.
π‘Example:
git branch
git branch -a
git branch -d <-branch-name->
git checkout
git checkout
switches branches or restores working tree files.
π οΈ Handy Options:
-b <branch>
: Creates a new branch and checks it out.
π‘Example:
git checkout <-branch->
git checkout -b <-new-branch->
git stash
The git stash
command temporarily shelves changes in the working directory, allowing you to switch branches or perform other operations without committing the changes. Stashing is useful when you need to work on something else urgently but don’t want to commit incomplete changes.
π οΈ Handy Options:
save
: Stashes changes with a custom message for reference.list
: Lists all stashed changes.apply
: Applies the most recent stash to the working directory without removing it from the stash list.pop
: Applies the most recent stash and removes it from the stash list.drop
: Removes a specific stash from the stash list.
π‘Example:
git stash save "Work in progress"
git stash list
git stash apply
git stash pop
git stash drop stash@{1}
Sharing and Updating Projects
git fetch
git fetch
retrieves changes from a remote repository, but it doesnβt integrate those changes into your working branch.
π‘Example:
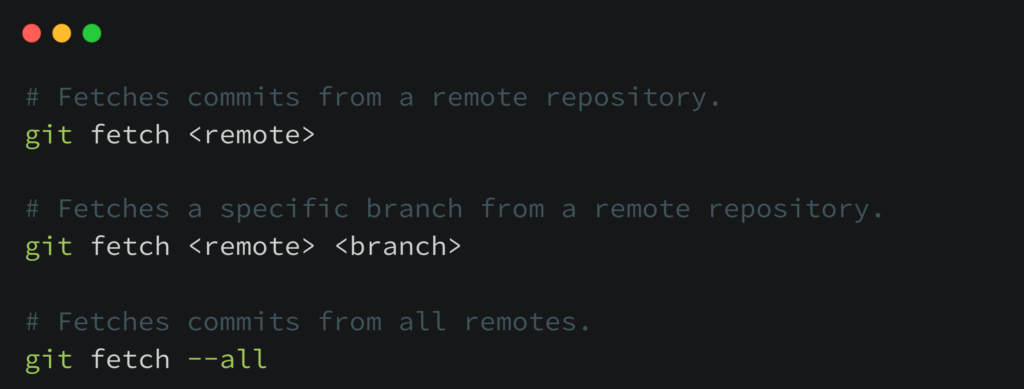
git pull
git pull
fetches changes from a remote repository and integrates them into the current branch.
π‘Example:
# Pulls changes from a remote repository and merges them.
git pull
# Pulls changes from a remote repository without committing.
git pull --no-commit
# Pulls changes from a remote repository and rebases local changes.
git pull --rebase
# Pulls changes from a remote repository with verbose output.
git pull --verbose
git push
git push
sends local commits to the remote repository.
π οΈ Handy Options:
-u <remote> <branch>
: Sets the upstream branch.
π‘Example:
# Pushes commits from a branch to a remote repository.
git push
# Pushes commits forcefully to overwrite conflicting changes.
git push --force
# Pushes all tags to the remote repository.
git push --tags
# Deletes a local and remote branch.
git branch -D branch_name
# Pushes the local 'main' branch to the remote and sets it as the upstream branch.
git push -u origin main
Inspection and Comparison
git show
git show
displays various types of objects in the repository, including commits, tags, and trees, along with the content changes.
π οΈ Handy Options:
<commit>
: Shows information about a specific commit.<file>
: Shows changes made to a specific file.
π‘Example:
git show <-commit->
git show <-file->
git log
The git log
command displays a detailed history of commits in the repository. It provides information such as commit messages, authors, dates, and commit hashes. This command is essential for tracking changes, understanding project evolution, and identifying specific commits.
π οΈ Handy Options:
--oneline
: Condenses each commit to a single line, providing a concise overview of the commit history.--graph
: Displays the commit history as a text-based graph, showing branching and merging visually.--since=<date>
: Shows commits more recent than the specified date.--author=<author>
: Filters commits based on the author’s name or email.
π‘Example:
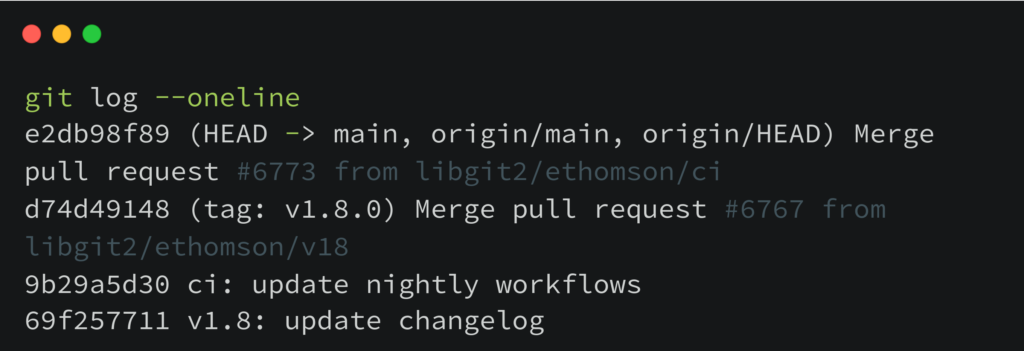
git log --graph
* commit e2db98f891485173470ee6763f5862af8ce4ab23 (HEAD -> main, origin/main, origin/HEAD)
|\ Merge: d74d49148 9b29a5d30
| | Author: Edward Thomson
| | Date: Fri Mar 22 10:44:36 2024 +0000
| |
| | Merge pull request #6773 from libgit2/ethomson/ci
| |
| | ci: update nightly workflows
| |
| * commit 9b29a5d30d1ea993498b9f9c91855ed0d56eea6a
| | Author: Edward Thomson
| | Date: Wed Mar 20 09:25:14 2024 +0000
| |
| | ci: update nightly workflows
| |
| | Update the nightly and benchmark workflows to only run steps in
| | libgit2/libgit2 by default. Also update the benchmark workflow to use
| | the latest download-artifact version.
| |
* | commit d74d491481831ddcd23575d376e56d2197e95910 (tag: v1.8.0)
# Displays the commit log since January 1st, 2023.
git log --since="2023-01-01"
# Displays the commit log authored by "John Doe".
git log --author="John Doe"
git diff
The git diff
command is used to view the differences between various states of your repository, such as changes between the working directory and the staging area, or between different commits. It provides a detailed breakdown of modifications, making it easier to understand the changes made to files.
π‘Example:
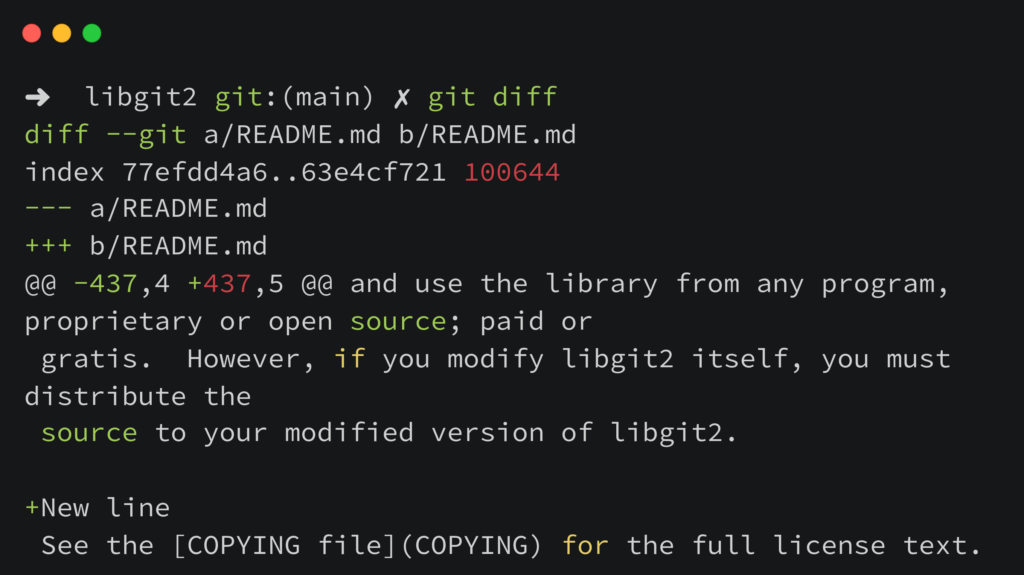
Patching
git apply
git apply
applies a patch to files in the working directory.
π οΈ Handy Options:
--check
: Checks if the patch can be applied cleanly without actually applying it.--3way
: Applies the patch using a three-way merge if it cannot be applied cleanly.
π‘Example:
git apply <-patch-file->
git apply --check <-patch-file->
git cherry-pick
The git cherry-pick
command is used to apply a specific commit from one branch to another. This is particularly useful when you need to incorporate a single commit from one branch into another branch without merging the entire branch.
π‘Example:
git cherry-pick <-commit-hash->
Debugging
git bisect
git bisect
helps find the commit that introduced a bug by using binary search.
π οΈ Handy Options:
start
: Starts the bisecting process.good <commit>
: Marks a commit as good.bad <commit>
: Marks a commit as bad.
π‘Example:
git bisect start
git bisect good <-commit->
git bisect bad <-commit->
Administration
git gc
git gc
cleans unnecessary files and optimizes the local repository.
π‘Example:
git gc
git reflog
The git reflog
command displays a log of reference changes in the repository, including commits and other updates to the HEAD. It provides a detailed history of recent actions, even if commits have been undone or branches deleted. This can be particularly useful for recovering lost commits or understanding changes in the repository’s state.
π‘Example:
git reflog
Conclusion
By adding these extra Git commands to your daily tasks, you’ll be better able to look over changes, make patches, fix bugs, handle contributions, and effectively run your Git repository.
Remember that the best way to learn these directions is to practice them. Try them out in different situations to learn more about version control and get better at using it.
Use the official Git documentation to find out more and read more specific instructions.
Got any queries or feedback? Feel free to drop a comment below!